5
Javascript Tutorial #1 - Basic Syntax & Operators
Zach Ripka @zachrip_
Javascript is a scripting language, it is used widely across the web and even in desktop programs to build applications. While Javascript is generally placed in an HTML(Hypertext Markup Language, what most web pages are made up of), it is it's own language. Javascript is not to be confused with Java, while there are a few similarities, Javascript is a scripting language, while Java is a compiled language. Javascript does not need to be compiled in order to run, it can be modified and run instantly. Let's get started with some basic Javascript, but first I need you to download the following:
- Google Chrome (https://www.google.com/chrome, you may not use it already, but if you use it for my tutorials, things will go easier)
- Komodo Edit (http://www.activestate.com/komodo-edit/downloads) OR Notepad++ (http://notepad-plus-plus.org/download/v6.3.3.html)
Once you have downloaded these two programs, let's get started. I'm going to start this tutorial off easy, we're just gonna output some text onto your screen and use a few operators.
Open Komodo Edit or NP++ and create a new file called "jtut1.html" (without the quotes) Be sure that the file is saved as an html file, or else this won't work right.
Alright, you've got your file open, I told you above that Javascript is generally placed within HTML, so let's get out basic HTML "shell" out of the way first. Since I want you to learn the syntax better, and remember the code that you're writing, and not just copy/paste mine, I'm going to place images of my source code, and you can copy it from the image.
Here is what you need to start with, it's what every HTML file starts with:

As you can see, there are the words "html" surrounded by angle brackets. These are called "tags" and they're part of HTML, not Javascript. The first tag "" is the opening tag, and the second tag, or closing tag is "." The top of every HTML file is either the opening HTML tag, or a "" tag, but don't worry about that for now. The last tag of every HTML file will always be the closing HTML tag.
Alright, next is the "head" tag, it contains metadata about the page, such as the page title (the text that displays on the tab of the site), the "favicon" (the icon next to the page title), and other things, such as keywords for search engines to read, and importing Javascript files.
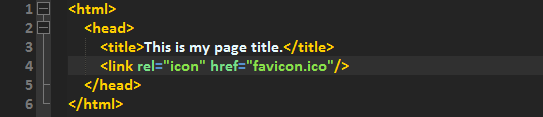
As you can see, there are three more tags added: "head," "title," and "link." I've already covered what the head tag is. I've also covered the title tag. But I'd like to explain the link tag further. The link tag is a way of linking to things, such as stylesheets, icons, and other things. This link tag I have is linking to the page icon, and the "href" parameter is the direct link to it. In this case, the html file and the icon would have to be in the same directory. You can also see that the filename ends in "ico," this can be any image type not just ico, for most browsers.
Alright, now let's add the body tag:
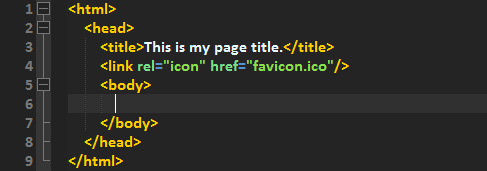
This is the body tag, it contains all of the actual page contents, such as these tags: paragraph, header, div, links, and images.
Now lets actually get into the Javascript. There are several ways you can include Javascript in an HTML page. Here is the first one (you don't actually need to code these):
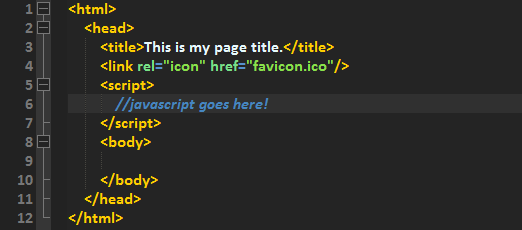
As you can see, your Javascript would go right into the script tags, within the head tags.
Here is another way:
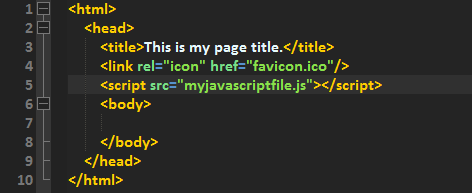
In this one, the Javascript script tags are still in the head tags, but it's just grabbing a Javascript file instead. All of your Javascript would be in "myjavascriptfile.js." Now both of these methods can also be used within the body tags, like this:
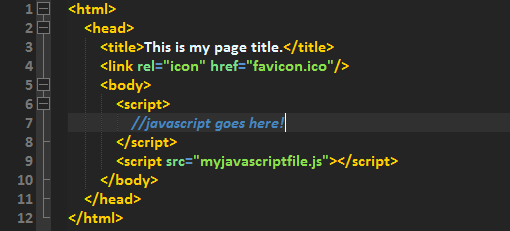
Alright, the method I usually use is the putting the script tags below all of the content in the body tags, this way there are no issues with the content not being loaded before my Javascript fires, removing any errors that could be cause by this. Let's get coding some Javascript. We're going to start by outputting our names, and a short message to the screen (you can start coding now):
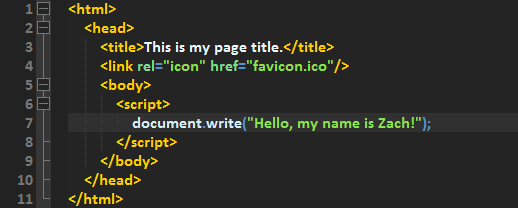
So you're probably confused: "what the heck is documawhwaha?" - Well, the way Javascript interacts with the page is through the DOM ("Document Object Model" http://en.wikipedia.org/wiki/Document_Object_Model). The page that you're interacting is called the DOM, and the overall top of the hierarchy is called "document." Now, what we're doing is calling the "write" method of "document," which, if we save our file and open it in our browser:
Here is the easy way to open your HTML file in Chrome
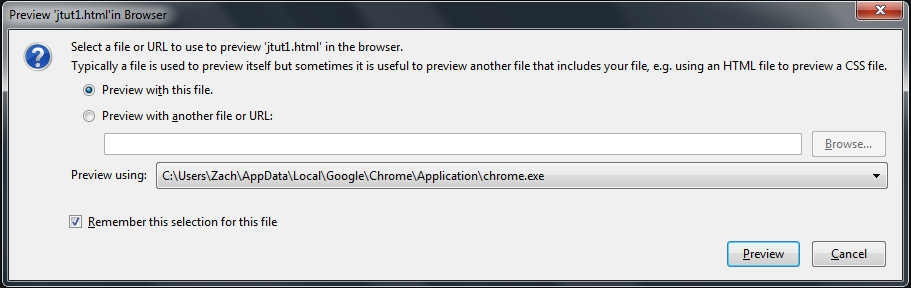
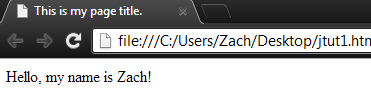
Yay, we've just successfully wrote our first Javascript! Now I'd like to introduce you into "vars," they're variables. This sounds hard, but it's just like in Math class when you replace "x" with a number. We're going to replace our name with the variable "name":
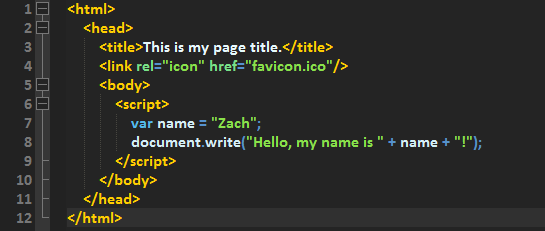
As you can see, we now have the variable "name" and we set it equal to our name. In Javascript, all strings must be surrounded in literals, or quotes (" or '). We also modified our document.write call to having a string, a variable, and another string. In between them, we put addition signs, which is basically like saying that you want to combine the three strings into one, then output it. Let's see it in our browser, reload that page you had open earlier.
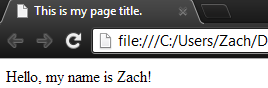
The same thing is outputted, just in a different way.
Let's add some numbers:
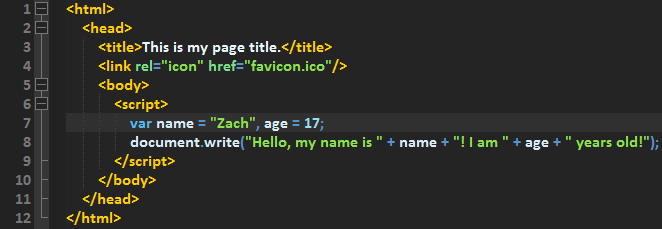
Now save and reload the page and we get:
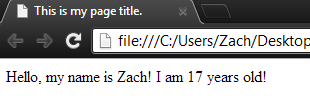
As you can see, numbers work the same as Strings, just without the quotes or literals. Also, instead of creating variables all with with same "var" you can do something like this: "var name = "Zach"; var age = 17;" But the way I did it is better.
Now let's use some basic operators, we can start with the four basics: addition, subtraction, division, and multiplication. Don't code all of the following ones, just look at the different way that the operators can be used (these will all output the same things that the last code example did):
Addition:
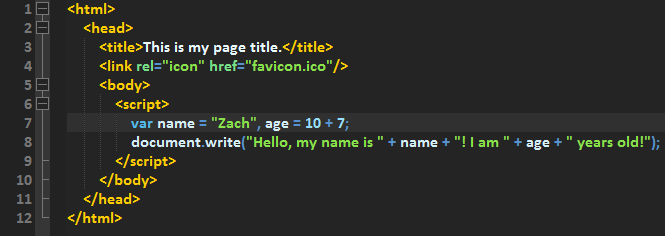
As you can see, the variable "age" is set to "10 + 7," so of course, "age" equals "17." Here is another way to accomplish the same output, but this time age will only equal 10, but we'll add 7 to it in the document.write call:
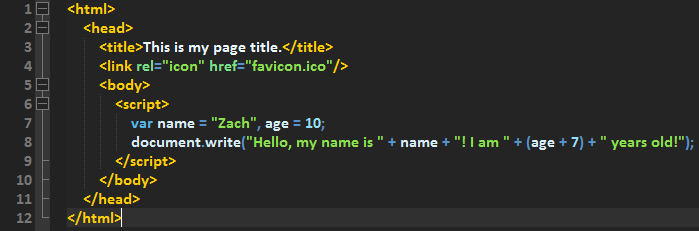
Alright, so we set "age" to 10, then in document.write we put parenthesis around age and 7, without the parenthesis, it'd output 107, instead of 17.
Subtraction:
This one is pretty self explanatory, but we're taking 24 - 7, and assigning that to the variable "age."
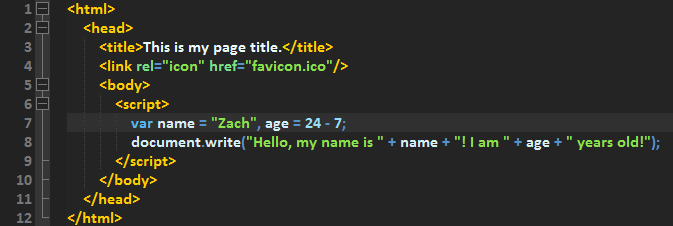
Same as addition, we can put the parenthesis around it, and do it in the document.write call instead:
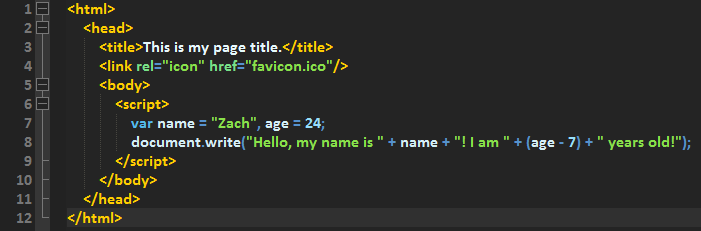
Multiplication:
Multiplication is used the same way as subtraction, or addition, but instead of using the normal Math "x means multiply," you use the Algebra "* means multiply" :
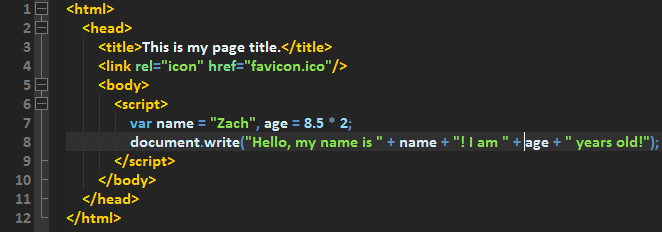
Same as the last two operators, you can do the math in the document.write call:
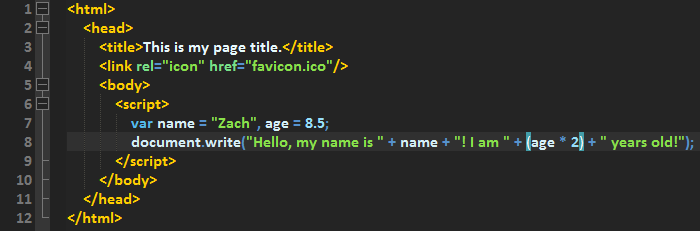
Division:
Division is the same as the rest of the operators above, but you use "/" to divide things:
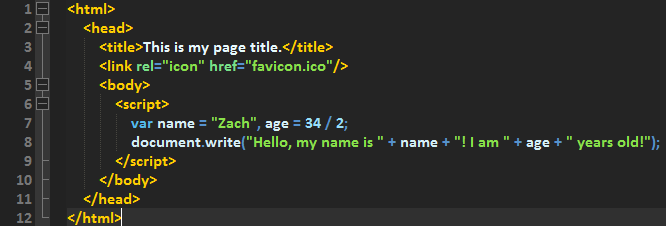
Same as all the other operators, we can do the operation in the document.write call, like this:
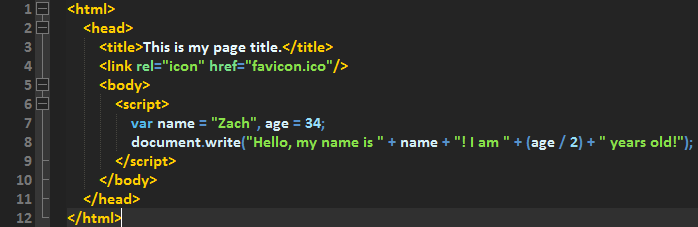
Alright, I've covered the basic four operators, now for the final two operators for today:
When used, this operator adds 1 to whatever the variable is currently at, like this:
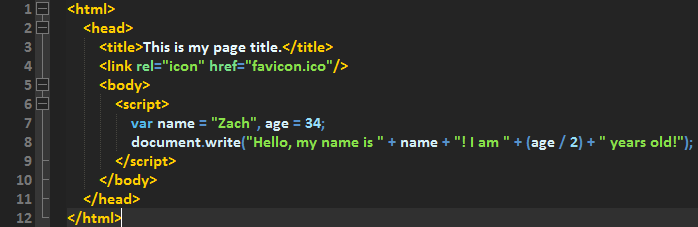
This would be the same as taking 16 + 1.
Here is the opposite:
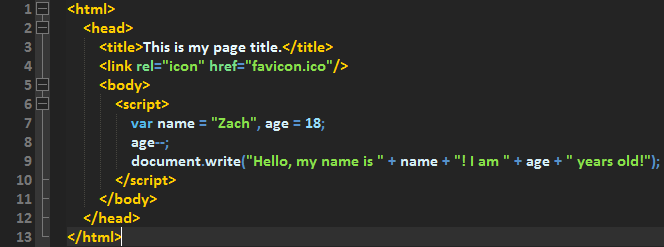
This would be the same as taking 18 - 1.
If you made it this far, congrats, you're at the end of the tutorial.
Be sure to check out my other blogs, I'll be posting nearly daily!
Don't forget to leave feedback below, and ask questions!
Tags |
2240204
6
Create an account or sign in to comment.
A good Suggestion would maybe just go further into Javascript or HTML individually